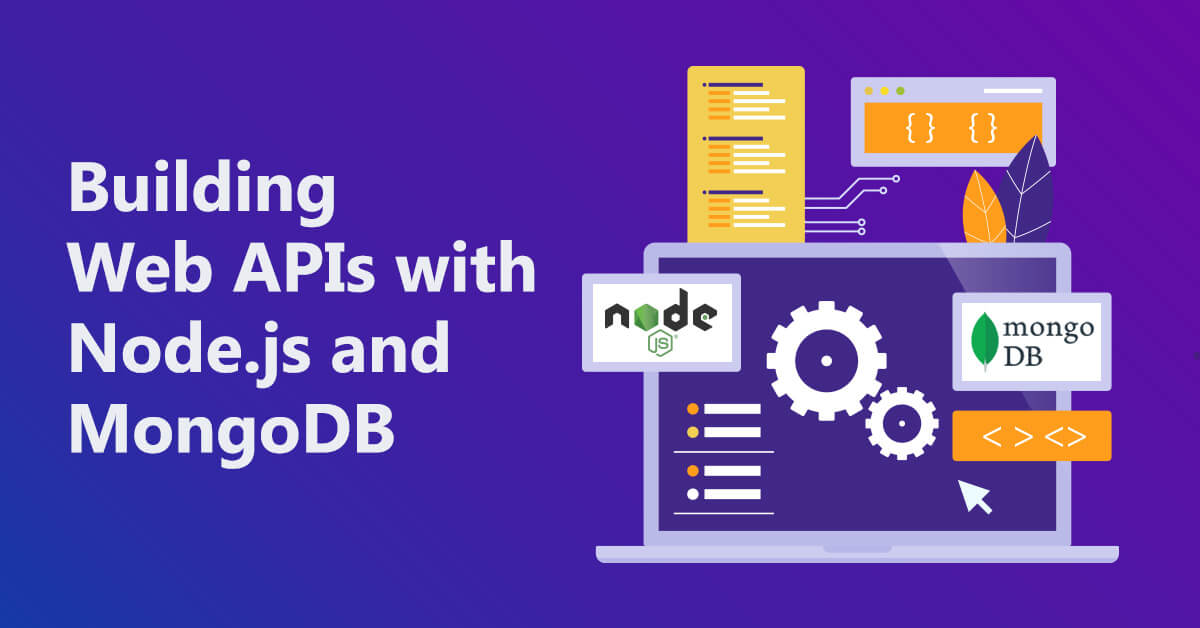
Application Programming Interfaces (API), are becoming increasingly prevalent these days. They are essential for executing data exchange and communication between services and servers. APIs allow applications to exchange critical information and details with one another. API services are also used to communicate with one another. Additionally, there are a large number of websites that use APIs to scale up. In order to meet this need, developers frequently express an interest in understanding how to create a bespoke API. This blog will instruct you on how to create web APIs using Node.JS and MongoDB, among other things.
What does NTSPL offer companies for Node.JS Solutions?
- Real-Time App Development
- Microservices Development
- Rich Portal Development
- Ecommerce Solutions
- Social Networking Application
- IoT-Based Application
- API Development For Mobile App Or Backend
- API Development And Integration
- Node.JS CMS Development
7 steps that will help you build web APIs by integrating MongoDB and Node.JS
In this post, you will learn how to develop a web API with MongoDB and Node.JS by following these technical steps.
Initialization of the Project
First of all, we will have to create an application folder for our application to reside. In order to start the application, we have to create a package.json file. This file will keep all the metadata required for the node application. This file enables npm to tackle the installation of package dependencies and the scripts that we would be writing to manage the application. ‘npm init –y’
Use this command to create the package.json file.
Installation of Application Dependencies
Further, we will require a file that serves as a command central for our application. Npm will first execute this file when you ask it to run the application. Know that this file will have third party modules which we install from the npm directory, as well as to object instances of multiple modules. All these modules serve as the dependencies of the project.
touch app.js
npm install express MongoDB body-parser –save
This command will be used to create a file named app.js in the current directory. This will serve as the primary point of access to the application’s functionality. Also required are the following dependencies, which must be installed:
- MongoDB: It is the official module of MongoDB and enables the Node.JS application to communicate with MongoDB.
- Body-parser: It will enable us to tackle request bodies with Express
- Express: It is a Node.JS framework.
Running the Application
We will have to run the code for our application. It will look like –
const Express = require(“express”);
const BodyParser = require(“body-parser”);
const MongoClient = require(“mongodb”).MongoClient;
const ObjectId = require(“mongodb”).ObjectID;
var app = Express();
app.use(BodyParser.json());
app.use(BodyParser.urlencoded({ extended: true }));
app.listen(5000, () => {});
In this process, we are basically importing the dependencies that we have downloaded earlier. We start with the Express framework by using the Express object. This, in turn, will help to initiate the server as well as run our application.
Testing the Application
In order to test the application, we will have to run the following boilerplate:
Node app.js
Establishing connection with MongoDB
Next, we will have to acquire the connection string with MongoDB. You will find this in the Atlas dashboard with the help of Clusters. Then go to the Overview tab and then onto the Connect button. Now we have to add the string to our app.js and come up with the below given changes to the code:
const Express = require(“express”);
const BodyParser = require(“body-parser”);
const MongoClient = require(“mongodb”).MongoClient;
const ObjectId = require(“mongodb”).ObjectID;
const CONNECTION_URL = ;
const DATABASE_NAME = “accounting_department”;
var app = Express();
app.use(BodyParser.json());
app.use(BodyParser.urlencoded({ extended: true }));
var database, collection;
app.listen(5000, () => {
MongoClient.connect(CONNECTION_URL, { useNewUrlParser: true }, (error, client) => {
if(error) {
throw error;
}
database = client.db(DATABASE_NAME);
collection = database.collection(“personnel”);
console.log(“Connected to `” + DATABASE_NAME + “`!”);
});
});
By using this code, we have basically defined the connection string that we need to use. Now you have to add a Connection_URL property. This depends on the connection string that you have received from MongoDB Atlas. Here we have also defined the name of the database which you want to create. The next time we start the application again, we can establish the connection. After that, we can use globally defined variables.
Next, we have to design out HTTP consumable API endpoints.
Building REST API Endpoints
We will now be developing endpoint for the creation and querying of the data. For this purpose, we will create an endpoint for adding the data. The below-given code needs to be added to app.js.
app.post(“/personnel”, (request, response) => {
collection.insert(request.body, (error, result) => {
if(error) {
return response.status(500).send(error);
}
response.send(result.result);
});
});
There is no need to do any data validation. In case a client creates a POST request towards the /personnel endpoint, we will insert the body into our collection. We will be returning the information back to the client depending on the error or successful response of the database.
Putting Things Together
Now that things are put together, we need to test our application.
curl -X POST \
-H ‘content-type:application/json’ \
-d ‘{“firstname”:”John”,”lastname”:”Doe”}’ \
http://localhost:5000/personnel
When you do not receive any errors, you will get to see the personal record for John Doe that has been added to MongoDB of the accounting_department. Now you can add multiple records easily to your database.
Now create an endpoint in order to retrieve the complete data records. For this purpose, use this code:
app.get(“/personnel”, (request, response) => {
collection.find({}).toArray((error, result) => {
if(error) {
return response.status(500).send(error);
}
response.send(result);
});
});
Our aim is to return all the data of our collection. If you add more records into your database then use the following endpoint to the app.js.
app.get(“/personnel/:id”, (request, response) => {
collection.findOne({ “_id”: new ObjectId(request.params.id) }, (error, result) => {
if(error) {
return response.status(500).send(error);
}
response.send(result);
});
});
Takeaway
This blog explains the intricate process of developing a custom API and thereby connecting it to MongoDB in order to fetch as well as manipulate the data. Once you gain mastery over these steps, try expanding the project using features such as data validation. Improving the API security can also be done by using Node.JS open-source platform.
NTSPL provides Full-stack development services based on the Node.JS open-source platform, which is one of the most popular technologies for developing scalable network applications more quickly than ever before.
Take advantage of NTSPL’s Node JS developers who have proven track records of delivering customer-centric Node JS application development solutions to a diverse spectrum of international clientele. Offering high-performance, business-oriented applications, NTSPL provides everything from app consulting to app implementation.