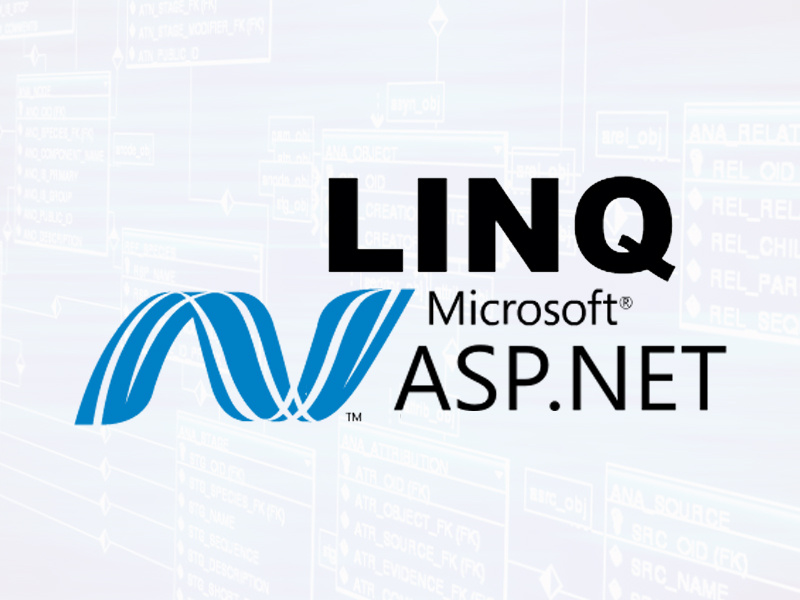
Language-Integrated Query (LINQ) was introduced in the .NET Framework 3.5. It features a unified model for querying any System.Collections.IEnumerable or System.Collections.Generic.IEnumerable<T> data source in a type-safe manner. LINQ to Objects is the name for LINQ queries that are run against in-memory collections such as List<T> and arrays. This article assumes that you have a basic understand of LINQ.
Parallel LINQ (PLINQ) is a parallel implementation of the LINQ pattern. A PLINQ query in many ways resembles a non-parallel LINQ to Objects query. PLINQ queries, just like sequential LINQ queries, operate on any in-memory IEnumerable or IEnumerable<T> data source, and have deferred execution, which means they do not begin executing until the query is enumerated. The primary difference is that PLINQ attempts to make full use of all the processors on the system. It does this by partitioning the data source into segments, and then executing the query on each segment on separate worker threads in parallel on multiple processors. In many cases, parallel execution means that the query runs significantly faster.
Demo:
Step 1: Create a asp.net page in 4.0 version
Step 2 : write the code in .cs fie
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Diagnostics;
public partial class TestPLinq : System.Web.UI.Page {
protected void Page_Load(object sender, EventArgs e) {
if (!IsPostBack) {
IEnumerable<int> rng = Enumerable.Range(1, 10000000);
var query = rng.Where(d => d % 1234567 == 0).Select(d => d);
Stopwatch sw = Stopwatch.StartNew();
foreach (var v in query) {
Response.Write(v+"<br/>");
}
Response.Write("Time in Normal LINq time: "+sw.ElapsedMilliseconds+" ms");
////Parallel
Response.Write("<br/>");
sw.Restart();
var query1 = rng.AsParallel().Where(d => d % 1234567 == 0).Select(d => d);
foreach (var v in query) {
Response.Write(v + "<br/>");
}
Response.Write("Time in Parallel time: " + sw.ElapsedMilliseconds + " ms");
}
}
}
Result:
For print 8 number different 18 sec
Happy Coding….